Task
This question manipulates one-dimensional and two-dimensional arrays. In part (a) you will write a method to reverse elements of a one-dimensional array. In parts (b) and (c) you will write methods to reverse elements of a twodimensional array.
(a) Consider the following incomplete ArrayUtil class, which contains a static reverseArray method.
Write the ArrayUtil method reverseArray. For example, if arr is the array {2,7,5,1,0}, the call to reverseArray changes arr to be {0,1,5,7,2}. Complete method reverseArray below.
/** Reverses elements of array arr.
* Precondition: arr.length > 0.
* Postcondition: The elements of arr have been reversed.
* @param arr the array to manipulate
*/
public static void reverseArray(int[] arr)
b) Consider the following incomplete Matrix class, which represents a twodimensional matrix of integers. Assume that the matrix contains at least one integer.
Write the Matrix method reverseAllRows. This method reverses the elements of each row. For example, if mat1 refers to a Matrix object, then the call mat1.reverseAllRows() will change the matrix as shown below.
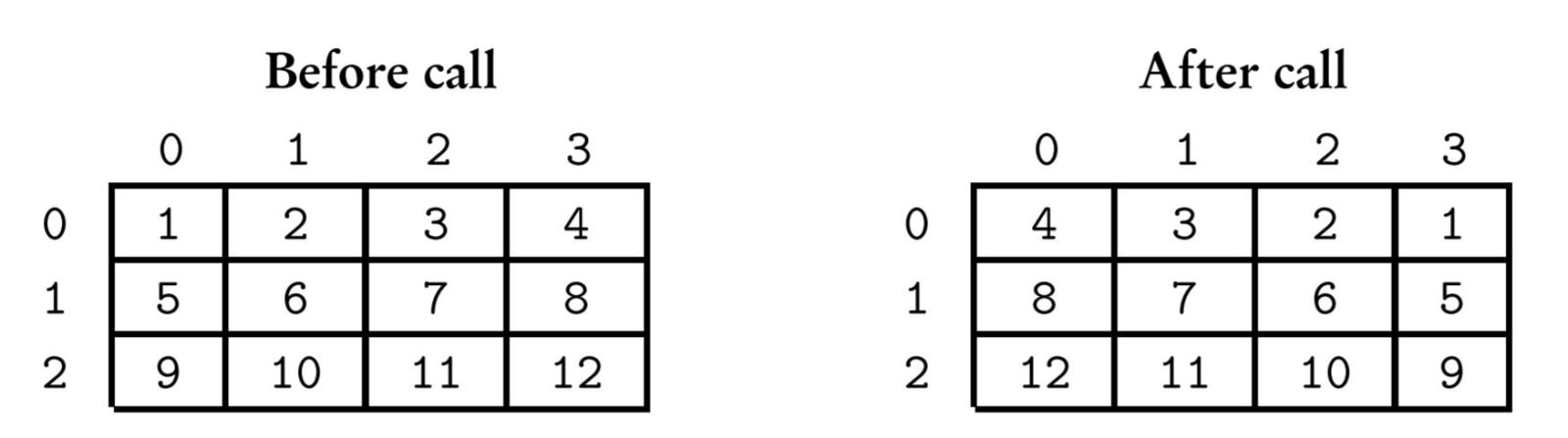
In writing reverseAllRows, you must call the reverseArray method in part (a). Assume that reverseArray works correctly regardless of what you wrote in part (a). Complete method reverseAllRows below.
/** Reverses the elements in each row of mat.
* Postcondition: The elements in each row have been reversed.
*/
public void reverseAllRows()
c) Write the Matrix method reverseMatrix. This method reverses the elements of a matrix such that the final elements of the matrix, when read in row-major order, are the same as the original elements when read from the bottom corner, right to left, going upward. Again let mat1 be a reference to a Matrix object. The the call mat1.reverseMatrix() will change the matrix as shown below.
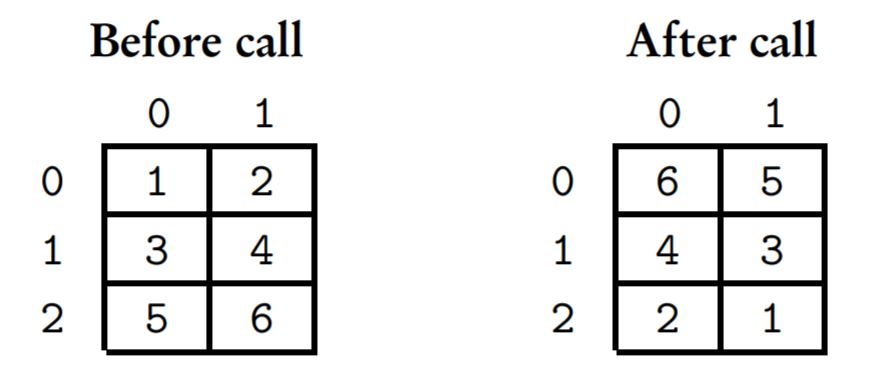
In writing reverseMatrix, you must call the reverseAllRows method in part (b). Assume that reverseAllRows works correctly regardless of what you wrote in part (b). Complete method reverseMatrix below.
/** Reverses the elements of mat.
* Postcondition:
* - The final elements of mat, when read in row-major order,
* are the same as the original elements of mat when read
* from the bottom corner, right to left, going upward.
* - mat[0][0] contains what was originally the last element.
* - mat[mat.length-1][mat[0].length-1] contains what was
* originally the first element.
*/
public void reverseMatrix()