Task
A text-editing program uses a Sentence class that manipulates a single sentence. A sentence contains letters, blanks, and punctuation. The first character in a sentence is a letter, and the last character is a punctuation mark. Any two words in the sentence are separated by a single blank. A partial implementation of the Sentence class is as follows.
a) Write the Sentencemethod getBlankPositions,which returns an ArrayList of integers that represent the positions in a sentence containing blanks. If there are no blanks in the sentence, getBlankPositions should return an empty list. Some results of calling getBlankPositions are shown below.
Complete method getBlankPositions below.
/** @return an ArrayList of integer positions containing a
* blank in this sentence. If there are no blanks in the
* sentence, returns an empty list.
*/
public List<Integer> getBlankPositions()
b) Write the Sentencemethod countWords,which returns the number of words in a sentence. Words are sequences of letters or punctuation, separated by a single blank. You may assume that every sentence contains at least one word.
For example:
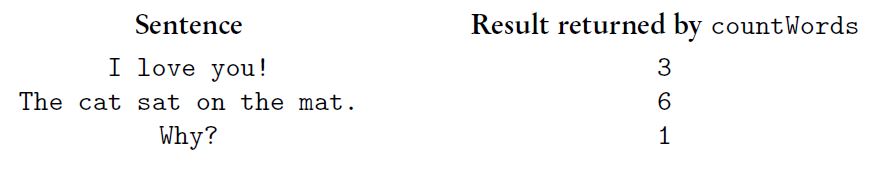
Complete method countWords below.
/** @return the number of words in this sentence
* Precondition: Sentence contains at least one word.
*/
public int countWords()
c) Write the Sentence method getWords, which returns an array of words in the sentence. A word is defined as a string of letters and punctuation, and does not contain any blanks. You may assume that a sentence contains at least one word.
Some examples of calling getWords are shown below.
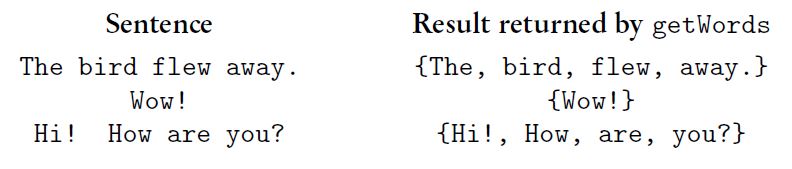
In writing method getWords, you must use methods getBlankPositions and countWords, which were written in parts (a) and (b). You may assume
that these methods work correctly, irrespective of what you wrote in parts (a) and (b).
Complete method getWords below.
/** @return the array of words in this sentence
* Precondition:
* - Any two words in the sentence are separated by one blank.
* - The sentence contains at least one word.
* Postcondition: String[] returned containing the words in
* this sentence.
*/
public String[] getWords()